Introduction – View Binding and Data Binding Android Kotlin
Android development has significantly evolved, offering developers powerful tools to create robust and efficient applications. Among these tools, View Binding and Data Binding stand out for their ability to simplify and enhance UI interactions. Both techniques are essential for developers building modern, maintainable, and high-performance Android applications. In this blog, we will delve deep into View Binding and Data Binding, their benefits, and differences, and how to implement them in your Kotlin-based Android projects effectively.
Table of Contents
What is View Binding?
View Binding is a feature introduced in Android Studio 3.6 that allows you to more easily write code that interacts with views. When you enable View Binding in a module, it generates a binding class for each XML layout file. An instance of the binding class contains direct references to all views that have an ID in the corresponding layout.

Benefits of View Binding
- Type Safety: View Binding generates classes that have direct references to views, eliminating the risk of null pointer exceptions.
- Compile-time Safety: Errors related to views are caught at compile-time rather than runtime.
- No More
findViewById()
: With View Binding, you can directly access the views without usingfindViewById()
, reducing boilerplate code. - Performance: View Binding is faster than other binding methods since it doesn’t require any parsing at runtime.
How to Enable View Binding
To enable View Binding in your project, add the following configuration to your build.gradle
file:
android {
...
viewBinding {
enabled = true
}
}
Once enabled, View Binding will generate a binding class for each XML layout file. For example, if you have a layout file named activity_main.xml
, View Binding will generate a class called ActivityMainBinding
.
Using View Binding in an Activity
Here’s how you can use View Binding in an Activity:
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.textView.text = "Hello, View Binding!"
}
}
Using View Binding in a Fragment
Using View Binding in a Fragment is slightly different but follows the same principle:
class MainFragment : Fragment() {
private var _binding: FragmentMainBinding? = null
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
_binding = FragmentMainBinding.inflate(inflater, container, false)
return binding.root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}
What is Data Binding?
Data Binding is a more powerful library introduced to Android to bind UI components in your layouts to data sources in your app using a declarative format rather than programmatically. Data Binding allows for a more flexible and scalable way to connect your UI components to your data model.
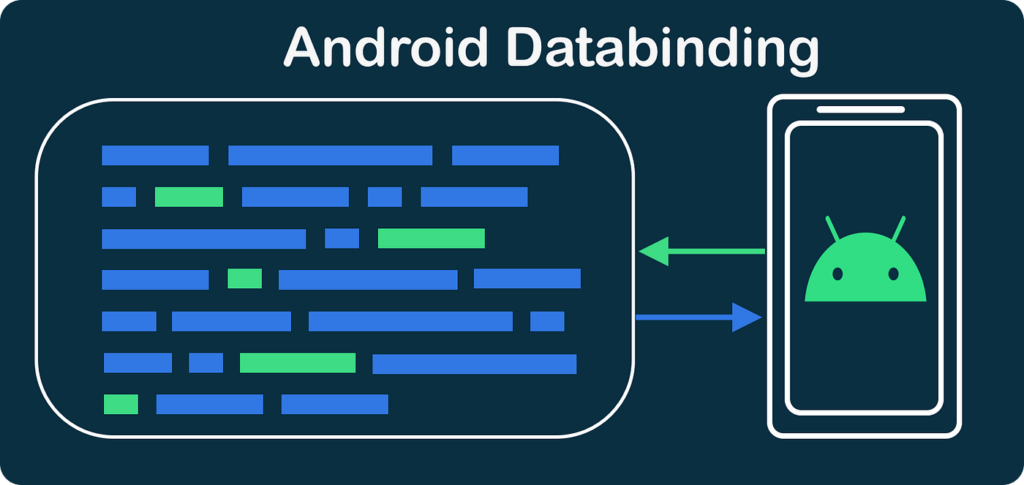
Benefits of Data Binding
- Less Boilerplate Code: Data Binding significantly reduces the boilerplate code required to set up UI interactions.
- Two-way Data Binding: Enables automatic synchronization between UI and data sources.
- Flexibility: Supports complex expressions, method references, and custom attributes.
- Lifecycle Awareness: Data Binding can be lifecycle-aware when used with LiveData and ViewModel, ensuring UI updates are in sync with the lifecycle.
How to Enable Data Binding
To enable Data Binding in your project, add the following configuration to your build.gradle
file:
android {
...
dataBinding {
enabled = true
}
}
Using Data Binding in an Activity
To use Data Binding, you need to update your XML layout file to be a binding layout. For example:
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable
name="viewModel"
type="com.example.app.MainViewModel" />
</data>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{viewModel.text}" />
</LinearLayout>
</layout>
In your Activity, you bind the layout and set the ViewModel:
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
private val viewModel: MainViewModel by viewModels()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = DataBindingUtil.setContentView(this, R.layout.activity_main)
binding.viewModel = viewModel
binding.lifecycleOwner = this
}
}
Two-way Data Binding
Two-way Data Binding is particularly useful when dealing with user inputs. To enable two-way Data Binding, use the @=
syntax in your XML:
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@={viewModel.inputText}" />
In your ViewModel, you should use MutableLiveData
to hold the input value:
View Binding vs. Data Binding
While both View Binding and Data Binding help reduce boilerplate code and improve UI interactions, they serve different purposes and have unique advantages:
- Complexity and Use Cases:
- View Binding: Simpler to set up and use, ideal for straightforward view interactions without the need for complex data synchronization.
- Data Binding: More powerful and flexible, suitable for scenarios requiring complex data bindings, two-way data binding, and integration with ViewModels and LiveData.
- Performance:
- View Binding: Generally faster since it doesn’t require XML parsing at runtime.
- Data Binding: May introduce a slight performance overhead due to its runtime parsing and more extensive feature set.
- Flexibility:
- View Binding: Limited to view references and direct interactions.
- Data Binding: Supports binding expressions, method references, custom attributes, and two-way data binding.
- Lifecycle Awareness:
- View Binding: Not lifecycle-aware.
- Data Binding: Can be made lifecycle-aware, ensuring UI updates are in sync with the component lifecycle.
Best Practices
- Use View Binding for Simple Views: For activities and fragments that don’t require complex data handling, prefer View Binding for its simplicity and performance benefits.
- Use Data Binding for Complex Interactions: For activities and fragments that involve complex data interactions, especially with ViewModels and LiveData, prefer Data Binding.
- Avoid Memory Leaks: In fragments, make sure to nullify the binding instance in
onDestroyView()
to avoid memory leaks. - Leverage Lifecycle Awareness: When using Data Binding with ViewModels and LiveData, set the lifecycle owner to ensure data updates are lifecycle-aware.
Conclusion
View Binding and Data Binding are powerful tools that can significantly streamline Android development with Kotlin. By understanding their unique features, benefits, and best use cases, you can make informed decisions about which approach to use in your projects. Whether you need the simplicity and performance of View Binding or the flexibility and power of Data Binding, these tools will help you build more efficient, maintainable, and high-quality Android applications.
By mastering View Binding and Data Binding, you equip yourself with the skills to create modern and responsive user interfaces, ultimately enhancing the overall user experience of your applications. Happy coding!