Introduction Firebase Firestore
Firebase Firestore is a powerful NoSQL document-oriented database that allows developers to store and sync data in real time across various platforms. In this guide, we will explore how to create a hierarchical data structure, such as a tree, using Firebase Firestore in a Kotlin-based Android application.
Table of Contents
NoSQL Databases
NoSQL databases are non-relational databases designed to provide flexible schemas, scalability, and high performance. They are often used to handle large volumes of unstructured, semi-structured, or structured data. Unlike traditional SQL databases, NoSQL databases do not use fixed table schemas and can store data in various formats, including key-value pairs, document-oriented, column-family, and graph formats.
Types of NoSQL Databases:
- Key-Value Stores: Data is stored as key-value pairs (e.g., Redis, DynamoDB).
- Document Stores: Data is stored in documents (e.g., JSON, BSON) and can be nested and complex (e.g., MongoDB, CouchDB).
- Column-Family Stores: Data is stored in columns rather than rows (e.g., Cassandra, HBase).
- Graph Databases: Data is stored as nodes, edges, and properties to represent relationships (e.g., Neo4j, ArangoDB).
Firebase Firestore
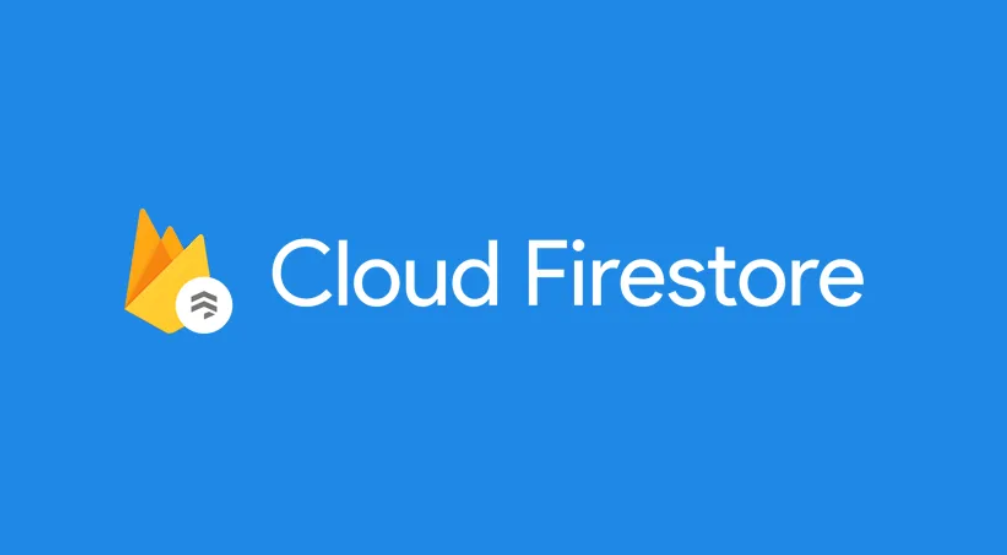
Firebase Firestore is a NoSQL document-oriented database provided by Google as part of its Firebase platform. It is designed to store and sync data for client- and server-side development. Firestore uses a document-collection model to structure data.
- Document: A document is a set of key-value pairs. It resembles a JSON object.
- Collection: A collection is a group of documents.
Tree Structure Databases
A Tree Structure Database represents data in a hierarchical format, similar to a tree with nodes and edges. Each node can have multiple child nodes but only one parent node, except for the root node, which has no parent. This structure is beneficial for representing hierarchical relationships like organizational structures, file systems, etc.
Setting Up Firebase Firestore
- Add Firebase Dependencies:
In your build.gradle
(app-level) file, add the following dependencies:
dependencies {
implementation platform('com.google.firebase:firebase-bom:31.2.0')
implementation 'com.google.firebase:firebase-firestore-ktx'
}
- Initialize Firebase in Your Application:
In your MainActivity.kt
or another suitable entry point, initialize Firestore:
package com.example.myfirebaseapp
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.google.firebase.firestore.FirebaseFirestore
import com.google.firebase.ktx.Firebase
import com.google.firebase.firestore.ktx.firestore
class MainActivity : AppCompatActivity() {
private lateinit var db: FirebaseFirestore
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Initialize Firestore
db = Firebase.firestore
// Create hierarchical data structure
createHierarchicalData()
}
private fun createHierarchicalData() {
val rootRef = db.collection("categories").document("root")
val childRef1 = rootRef.collection("subcategories").document("child1")
val childRef2 = rootRef.collection("subcategories").document("child2")
val rootData = hashMapOf(
"name" to "Root Category"
)
val childData1 = hashMapOf(
"name" to "Child Category 1"
)
val childData2 = hashMapOf(
"name" to "Child Category 2"
)
// Add root document
rootRef.set(rootData)
.addOnSuccessListener {
// Add child documents
childRef1.set(childData1)
childRef2.set(childData2)
}
.addOnFailureListener { e ->
// Handle the error
}
}
}
Reading the Hierarchical Data
To read the hierarchical data from Firestore:
private fun readHierarchicalData() {
val rootRef = db.collection("categories").document("root")
rootRef.get()
.addOnSuccessListener { document ->
if (document != null) {
val rootName = document.getString("name")
println("Root: $rootName")
// Reading subcategories
rootRef.collection("subcategories").get()
.addOnSuccessListener { subcategories ->
for (subcategory in subcategories) {
println("Subcategory: ${subcategory.getString("name")}")
}
}
}
}
.addOnFailureListener { e ->
// Handle the error
}
}
Adding Nested Data
If you want to add more nested levels to your tree structure, you can extend the existing structure:
private fun addNestedData() {
val rootRef = db.collection("categories").document("root")
val childRef1 = rootRef.collection("subcategories").document("child1")
val grandchildRef1 = childRef1.collection("subsubcategories").document("grandchild1")
val grandchildData1 = hashMapOf(
"name" to "Grandchild Category 1"
)
grandchildRef1.set(grandchildData1)
.addOnSuccessListener {
println("Grandchild category added successfully!")
}
.addOnFailureListener { e ->
println("Error adding grandchild category: ${e.message}")
}
}
Updating Documents
To update a document, you can use the update()
method:
private fun updateDocument() {
val childRef1 = db.collection("categories").document("root")
.collection("subcategories").document("child1")
childRef1.update("name", "Updated Child Category 1")
.addOnSuccessListener {
println("Document successfully updated!")
}
.addOnFailureListener { e ->
println("Error updating document: ${e.message}")
}
}
Deleting Documents
To delete a document, you can use the delete()
method:
private fun deleteDocument() {
val childRef1 = db.collection("categories").document("root")
.collection("subcategories").document("child1")
childRef1.delete()
.addOnSuccessListener {
println("Document successfully deleted!")
}
.addOnFailureListener { e ->
println("Error deleting document: ${e.message}")
}
}
Querying Documents
You can query documents based on certain criteria. For example, to retrieve all subcategories of a root category:
private fun querySubcategories() {
val rootRef = db.collection("categories").document("root")
rootRef.collection("subcategories").whereEqualTo("name", "Child Category 1").get()
.addOnSuccessListener { documents ->
for (document in documents) {
println("Subcategory: ${document.getString("name")}")
}
}
.addOnFailureListener { e ->
println("Error querying documents: ${e.message}")
}
}
Handling Nested Data Structures
When working with deeply nested data structures, it’s often useful to structure your data model accordingly. Here’s an example of a function that adds a complete hierarchical structure:
private fun addCompleteTreeStructure() {
val rootRef = db.collection("categories").document("root")
val rootData = hashMapOf("name" to "Root Category")
val childData = listOf(
hashMapOf("name" to "Child Category 1"),
hashMapOf("name" to "Child Category 2")
)
val grandchildData = listOf(
hashMapOf("name" to "Grandchild Category 1"),
hashMapOf("name" to "Grandchild Category 2")
)
rootRef.set(rootData)
.addOnSuccessListener {
for (i in childData.indices) {
val childRef = rootRef.collection("subcategories").document("child${i + 1}")
childRef.set(childData[i])
.addOnSuccessListener {
for (j in grandchildData.indices) {
val grandchildRef = childRef.collection("subsubcategories").document("grandchild${j + 1}")
grandchildRef.set(grandchildData[j])
}
}
}
}
.addOnFailureListener { e ->
println("Error adding root category: ${e.message}")
}
}
Listening for Real-time Updates
Firestore provides real-time updates. You can listen to changes in your documents or collections:
private fun listenForUpdates() {
val rootRef = db.collection("categories").document("root")
rootRef.addSnapshotListener { snapshot, e ->
if (e != null) {
println("Listen failed: ${e.message}")
return@addSnapshotListener
}
if (snapshot != null && snapshot.exists()) {
println("Current data: ${snapshot.data}")
} else {
println("Current data: null")
}
}
val subcategoriesRef = rootRef.collection("subcategories")
subcategoriesRef.addSnapshotListener { snapshots, e ->
if (e != null) {
println("Listen failed: ${e.message}")
return@addSnapshotListener
}
for (docChange in snapshots!!.documentChanges) {
when (docChange.type) {
DocumentChange.Type.ADDED -> println("New subcategory: ${docChange.document.data}")
DocumentChange.Type.MODIFIED -> println("Modified subcategory: ${docChange.document.data}")
DocumentChange.Type.REMOVED -> println("Removed subcategory: ${docChange.document.data}")
}
}
}
}
Conclusion
By following the steps outlined in this guide, you can create and manage hierarchical data structures in Firebase Firestore using Kotlin for your Android applications. This approach leverages Firestore’s document and collection model to represent complex relationships, making it a powerful tool for developing scalable and flexible applications.