In the world of Android development, managing concurrent tasks efficiently is a crucial aspect of building responsive and smooth applications. One of the most robust and versatile tools for handling concurrency in Android is the Executor framework. Executors provide a high-level API for managing threads, making it easier to run tasks in the background without getting bogged down by the complexities of thread management. In this blog, we’ll delve deep into Executors, exploring their importance, how to use them, and best practices for managing concurrent tasks in Kotlin for Android.
Introduction to Executors – Managing Concurrent Tasks
Table of Contents
What are Executors?
Executors are part of the java.util.concurrent
package introduced in Java 5. They provide a higher-level replacement for the traditional thread creation and management, allowing developers to focus more on the tasks to be executed rather than on the low-level details of thread management. Executors handle the creation, scheduling, and execution of tasks using a pool of worker threads, optimizing resource usage and improving application performance.
Why Use Executors in Android?
- Simplified Thread Management: Executors abstract away the complexity of creating and managing threads directly.
- Efficient Resource Utilization: By reusing a pool of threads, Executors reduce the overhead associated with thread creation and destruction.
- Scalability: Executors can handle a large number of tasks efficiently by queuing tasks and executing them as threads become available.
- Thread Safety: Executors provide built-in mechanisms to handle thread safety, reducing the risk of concurrency issues.
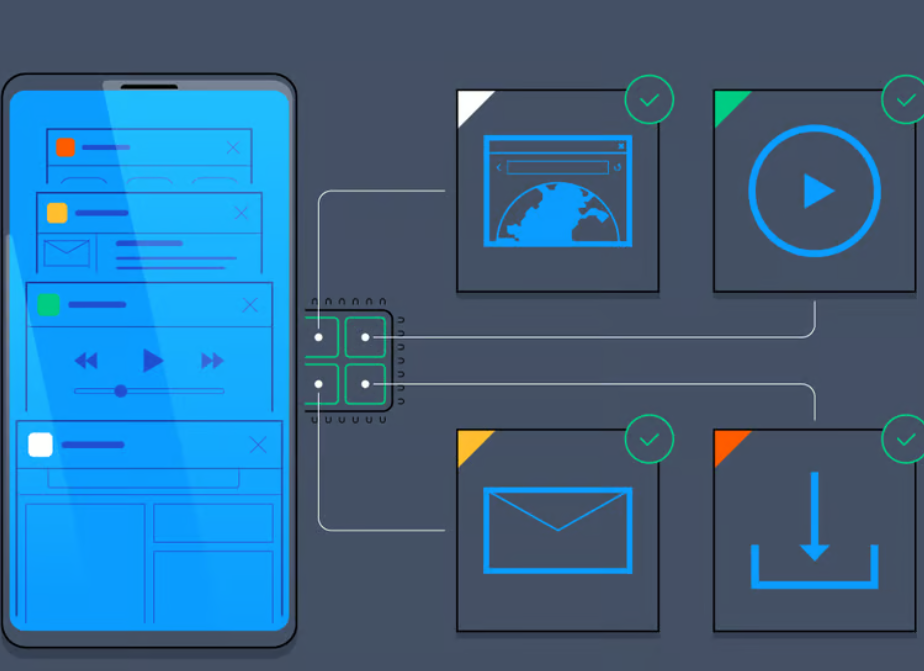
Types of Executors
There are several types of Executors available, each suitable for different use cases:
- SingleThreadExecutor: An Executor that uses a single worker thread to execute tasks sequentially.
- FixedThreadPool: An Executor that uses a fixed number of threads to execute tasks.
- CachedThreadPool: An Executor that creates new threads as needed but will reuse previously constructed threads when available.
- ScheduledThreadPool: An Executor that can schedule commands to run after a given delay or to execute periodically.
SingleThreadExecutor
The SingleThreadExecutor
is ideal for tasks that need to be executed sequentially. It ensures that tasks are executed one after another in the order they are submitted.
val executor = Executors.newSingleThreadExecutor()
executor.execute {
// Task 1
}
executor.execute {
// Task 2
}
FixedThreadPool
The FixedThreadPool
is suitable for executing a fixed number of tasks concurrently. It reuses a set number of threads to execute tasks.
val executor = Executors.newFixedThreadPool(4)
for (i in 1..10) {
executor.execute {
// Task $i
}
}
CachedThreadPool
The CachedThreadPool
dynamically adjusts the number of threads according to the number of tasks. It’s useful for executing many short-lived tasks.
val executor = Executors.newCachedThreadPool()
for (i in 1..10) {
executor.execute {
// Task $i
}
}
ScheduledThreadPool
The ScheduledThreadPool
allows you to schedule tasks to run after a delay or periodically.
val executor = Executors.newScheduledThreadPool(2)
executor.schedule({
// Task to run after 1 second
}, 1, TimeUnit.SECONDS)
executor.scheduleAtFixedRate({
// Task to run every 1 second
}, 0, 1, TimeUnit.SECONDS)
Using Executors in Android
Integrating Executors in an Android App
To demonstrate how to use Executors in an Android application, let’s create a simple example where we perform a background task to fetch data from a network and update the UI.
Step 1: Add Dependencies
Ensure you have the necessary dependencies in your build.gradle
file:
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
implementation 'androidx.appcompat:appcompat:1.3.0'
implementation 'androidx.core:core-ktx:1.6.0'
}
Step 2: Define the UI
Create a simple layout with a Button
and a TextView
in res/layout/activity_main.xml
:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<Button
android:id="@+id/buttonFetchData"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fetch Data" />
<TextView
android:id="@+id/textViewData"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Data will appear here" />
</LinearLayout>
Step 3: Implement the Logic in MainActivity
In your MainActivity.kt
, set up the Executor and perform the network request:
import android.os.Bundle
import android.os.Handler
import android.os.Looper
import androidx.appcompat.app.AppCompatActivity
import java.util.concurrent.Executors
class MainActivity : AppCompatActivity() {
private val executor = Executors.newSingleThreadExecutor()
private val mainHandler = Handler(Looper.getMainLooper())
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val buttonFetchData = findViewById<Button>(R.id.buttonFetchData)
val textViewData = findViewById<TextView>(R.id.textViewData)
buttonFetchData.setOnClickListener {
executor.execute {
val data = fetchDataFromNetwork()
mainHandler.post {
textViewData.text = data
}
}
}
}
private fun fetchDataFromNetwork(): String {
// Simulate network delay
Thread.sleep(2000)
return "Fetched Data"
}
}
n this example, we create a SingleThreadExecutor
to handle the network request. We use a Handler
associated with the main thread to update the UI once the data is fetched.
Best Practices for Using Executors
Choose the Right Executor: Select the appropriate type of Executor based on your use case. For tasks that should run sequentially, use SingleThreadExecutor
. For tasks that can run concurrently, use FixedThreadPool
or CachedThreadPool
.
Avoid Blocking the Main Thread: Always perform long-running tasks on a background thread to avoid blocking the main UI thread, which can lead to an unresponsive UI.
Graceful Shutdown: Ensure that you shut down the Executor properly when it’s no longer needed to free up resources. Use shutdown()
or shutdownNow()
methods.
Handle Exceptions: Handle exceptions within the tasks submitted to Executors to prevent the application from crashing.
Thread Safety: Ensure that shared resources are accessed in a thread-safe manner to avoid race conditions and data corruption.
Advanced Usage: Custom Thread Pools
In some cases, you may need more control over the behavior of the thread pool. You can create a custom ThreadPoolExecutor
to configure the core pool size, maximum pool size, keep-alive time, and task queue.
import java.util.concurrent.LinkedBlockingQueue
import java.util.concurrent.ThreadPoolExecutor
import java.util.concurrent.TimeUnit
val customExecutor = ThreadPoolExecutor(
2, // Core pool size
4, // Maximum pool size
60, // Keep alive time
TimeUnit.SECONDS,
LinkedBlockingQueue<Runnable>() // Task queue
)
for (i in 1..10) {
customExecutor.execute {
// Custom task
}
}
This approach provides fine-grained control over the thread pool, allowing you to tailor it to the specific needs of your application.
Conclusion
Executors are a powerful and flexible tool for managing concurrent tasks in Android applications. By abstracting away the complexities of thread management, Executors allow you to focus on building responsive and efficient applications. Whether you need to perform simple background tasks or manage a complex set of concurrent operations, Executors provide the tools you need to get the job done effectively.
Remember to choose the appropriate type of Executor for your use case, handle exceptions properly, and shut down Executors gracefully to maintain optimal performance and resource utilization. With these best practices, you’ll be well-equipped to manage concurrency in your Android applications using Kotlin and Executors.