Monetizing your Android app is a crucial step towards making your app development venture profitable. One of the most common ways to achieve this is through ad integration. Ad networks like AdMob and others provide a simple way to generate revenue by displaying ads within your app. This comprehensive guide will walk you through the process of integrating ads in your Android application using Kotlin, focusing primarily on AdMob, but also touching upon other ad networks.
Why Ad Integration in Android App?
Before diving into the technical details, let’s understand why you might want to integrate ads in your app:
- Revenue Generation: Ads are a significant source of income for many free apps. They allow you to provide your app for free while still generating revenue.
- User Engagement: Some ad formats, like rewarded video ads, can actually enhance user engagement by providing incentives for viewing ads.
- Cross-Promotion: Ads can be used to promote other apps you’ve developed, increasing visibility and downloads.
Table of Contents
Introduction to AdMob
AdMob is one of the most popular ad networks for mobile apps. It is owned by Google and offers a variety of ad formats, including banner ads, interstitial ads, rewarded video ads, and native ads. Integrating AdMob into your Android app using Kotlin is straightforward, thanks to its well-documented SDK and integration guides.
Getting Started with AdMob
To integrate AdMob in your Android app, follow these steps:
- Create an AdMob Account: If you don’t already have one, create an account on the AdMob website.
- Create a New AdMob App: In your AdMob account, add a new app by providing the necessary details.
- Get Ad Unit IDs: For each ad format you want to use, create an ad unit and get its unique ID. You’ll need this ID to load ads in your app.
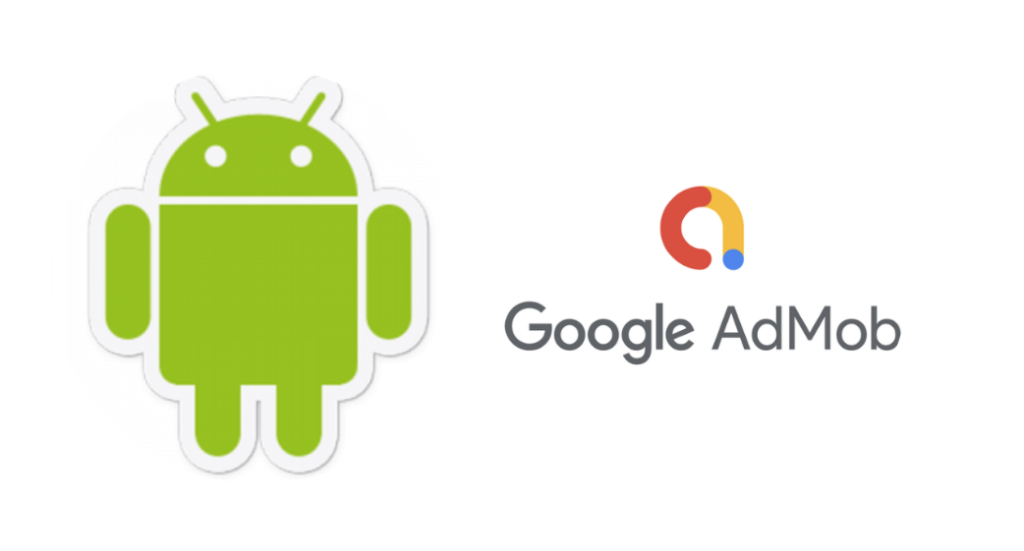
Adding AdMob to Your Android Project
- Add Dependencies: Open your
build.gradle
(app-level) file and add the necessary dependencies for AdMob.
dependencies {
implementation 'com.google.android.gms:play-services-ads:20.6.0'
}
2. Initialize the Mobile Ads SDK: In your application class or main activity, initialize the Mobile Ads SDK.
import com.google.android.gms.ads.MobileAds
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
MobileAds.initialize(this) {}
}
}
Implementing Different Ad Formats
Banner Ads
Banner ads are rectangular ads that occupy a portion of the screen. They are usually displayed at the top or bottom of the screen.
- Add AdView to Layout: In your XML layout file, add an
AdView
.
<com.google.android.gms.ads.AdView
android:id="@+id/adView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
ads:adSize="BANNER"
ads:adUnitId="ca-app-pub-3940256099942544/6300978111" />
2. Load the Banner Ad: In your activity, load the ad.
import com.google.android.gms.ads.AdRequest
import com.google.android.gms.ads.AdView
class MainActivity : AppCompatActivity() {
private lateinit var adView: AdView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
adView = findViewById(R.id.adView)
val adRequest = AdRequest.Builder().build()
adView.loadAd(adRequest)
}
}
Interstitial Ads
Interstitial ads are full-screen ads that cover the interface of the app until closed by the user.
- Load an Interstitial Ad: Load the ad in your activity.
import com.google.android.gms.ads.interstitial.InterstitialAd
import com.google.android.gms.ads.interstitial.InterstitialAdLoadCallback
class MainActivity : AppCompatActivity() {
private var interstitialAd: InterstitialAd? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val adRequest = AdRequest.Builder().build()
InterstitialAd.load(this, "ca-app-pub-3940256099942544/1033173712", adRequest, object : InterstitialAdLoadCallback() {
override fun onAdLoaded(ad: InterstitialAd) {
interstitialAd = ad
}
override fun onAdFailedToLoad(adError: LoadAdError) {
interstitialAd = null
}
})
}
private fun showInterstitial() {
interstitialAd?.show(this)
}
}
Rewarded Video Ads
Rewarded video ads provide users with incentives, such as in-app currency or extra lives, for watching video ads.
- Load a Rewarded Video Ad: Load the ad in your activity.
import com.google.android.gms.ads.rewarded.RewardItem
import com.google.android.gms.ads.rewarded.RewardedAd
import com.google.android.gms.ads.rewarded.RewardedAdLoadCallback
class MainActivity : AppCompatActivity() {
private var rewardedAd: RewardedAd? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val adRequest = AdRequest.Builder().build()
RewardedAd.load(this, "ca-app-pub-3940256099942544/5224354917", adRequest, object : RewardedAdLoadCallback() {
override fun onAdLoaded(ad: RewardedAd) {
rewardedAd = ad
}
override fun onAdFailedToLoad(adError: LoadAdError) {
rewardedAd = null
}
})
}
private fun showRewardedAd() {
rewardedAd?.show(this) { rewardItem: RewardItem ->
val rewardAmount = rewardItem.amount
val rewardType = rewardItem.type
// Reward the user.
}
}
}
Other Ad Networks
While AdMob is a popular choice, there are several other ad networks you might consider:
- Facebook Audience Network: Offers various ad formats and advanced targeting options.
- Unity Ads: Particularly useful for game developers, offering video ads and rewarded video ads.
- AppLovin: Provides a comprehensive ad platform with a wide range of ad formats.
- IronSource: Known for its mediation capabilities, allowing you to integrate multiple ad networks easily.
Integrating Facebook Audience Network
To integrate Facebook Audience Network in your app:
- Add Dependencies: Update your
build.gradle
(app-level) file.
dependencies {
implementation 'com.facebook.android:audience-network-sdk:6.5.1'
}
2. Initialize the SDK: In your application class or main activity, initialize the SDK
import com.facebook.ads.AudienceNetworkAds
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
AudienceNetworkAds.initialize(this)
}
}
3. Implement Banner Ads: Add a com.facebook.ads.AdView
to your layout.
<com.facebook.ads.AdView
android:id="@+id/adView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
ads:adSize="BANNER_HEIGHT_50"
ads:placementId="YOUR_PLACEMENT_ID" />
In your activity, load the ad.
import com.facebook.ads.Ad
import com.facebook.ads.AdError
import com.facebook.ads.AdListener
import com.facebook.ads.AdView
class MainActivity : AppCompatActivity() {
private lateinit var adView: AdView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
adView = findViewById(R.id.adView)
adView.loadAd(adView.buildLoadAdConfig().withAdListener(object : AdListener {
override fun onError(ad: Ad?, adError: AdError?) {
// Ad error callback
}
override fun onAdLoaded(ad: Ad?) {
// Ad loaded callback
}
override fun onAdClicked(ad: Ad?) {
// Ad clicked callback
}
override fun onLoggingImpression(ad: Ad?) {
// Ad impression logged callback
}
}).build())
}
}
Best Practices for Ad Integration
- User Experience: Ensure ads do not interfere with the user experience. Avoid placing ads in a way that disrupts the flow of your app.
- Ad Placement: Strategically place ads where they are visible but not intrusive. Banner ads are typically placed at the bottom of the screen.
- Ad Frequency: Be mindful of ad frequency to prevent user frustration. Too many ads can lead to a poor user experience and uninstalls.
- Testing: Thoroughly test ads on different devices to ensure they load correctly and do not cause crashes or performance issues.
Conclusion
Integrating ads into your Android app using Kotlin can be a straightforward process if you follow the steps outlined above. AdMob is an excellent choice for beginners due to its comprehensive documentation and support. However, exploring other ad networks like Facebook Audience Network, Unity Ads, AppLovin, and IronSource can provide additional monetization opportunities and benefits.
Remember to prioritize user experience and strategically place ads to maximize revenue without alienating your user base. With the right approach, ad integration can significantly boost your app’s profitability while maintaining a positive user experience.