Introduction to Espresso: In the world of Android development, testing is a crucial part of ensuring the quality and reliability of your applications. Among the various testing frameworks available, Espresso stands out as a powerful tool for UI testing. Developed by Google, Espresso allows developers to write concise and reliable UI tests for Android applications. In this blog, we’ll dive deep into Espresso, explore its features, and demonstrate how to use it effectively with Kotlin.
Table of Contents
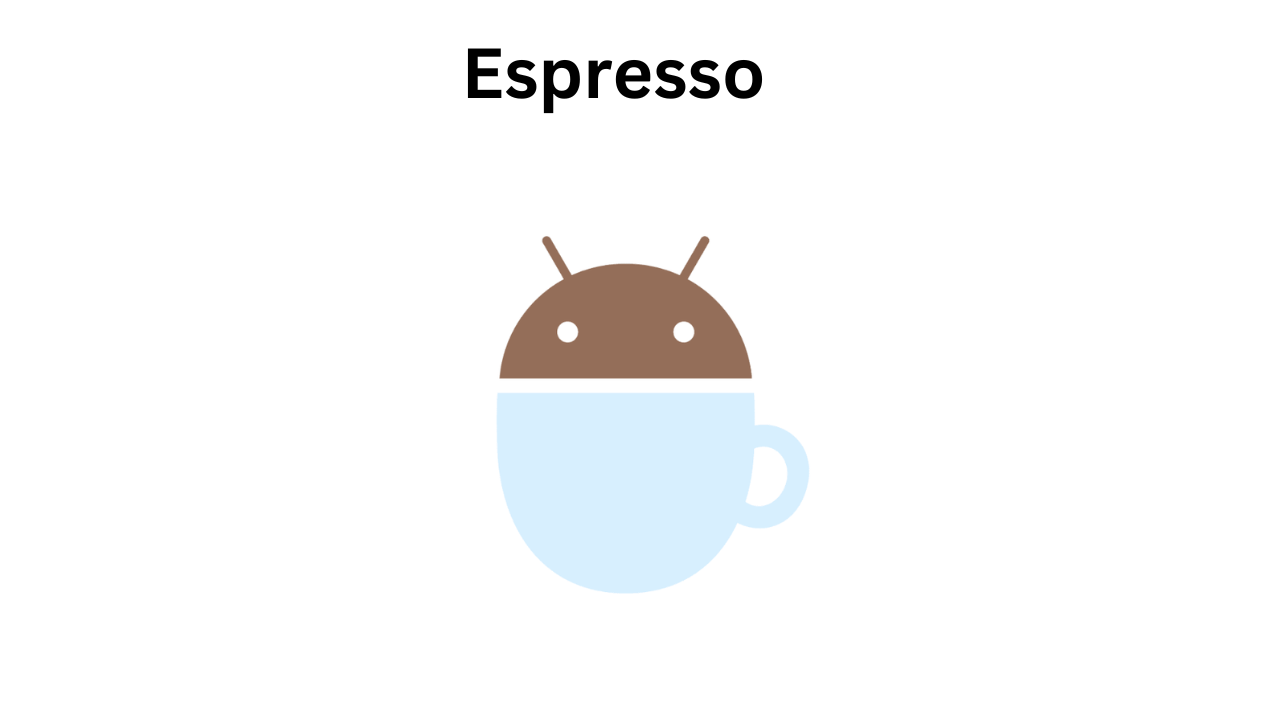
Why Use Espresso? : Introduction to Espresso
- Simplicity: Espresso’s API is straightforward to understand, making it accessible even for developers who are new to testing.
- Synchronization: Espresso automatically handles synchronization with the UI thread, reducing the chances of flaky tests.
- Flexibility: It supports both unit and functional UI testing, allowing you to test individual components as well as complete user flows.
- Integration: Espresso integrates seamlessly with Android Studio and other testing frameworks like JUnit, making it easy to incorporate into your existing development workflow.
Setting Up Espresso in Your Android Project
Before you start writing tests with Espresso, you need to set up your Android project. Here’s a step-by-step guide to get you started:
- Add Dependencies: Open your
build.gradle
file (usually the one in theapp
module) and add the following dependencies:
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test:rules:1.4.0'
2. Enable MultiDex: If your application is large and requires MultiDex, make sure it is enabled in your build.gradle
:
android {
defaultConfig {
multiDexEnabled true
}
}
3. Sync Your Project: After adding the dependencies, sync your project with Gradle to download the necessary libraries.
Writing Your First Espresso Test
Now that your project is set up, let’s write a simple Espresso test. We’ll create a basic app with a button and a text view. When the button is clicked, the text view will display a message. Our test will verify this behavior.
- Create a New Activity: Create a new activity named
MainActivity
with the following layout:
<!-- res/layout/activity_main.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="" />
</LinearLayout>
2. Implement the Activity: In your MainActivity.kt
, add the following code:
// src/main/java/com/example/espresso/MainActivity.kt
package com.example.espresso
import android.os.Bundle
android.widget.Button
android.widget.TextView
androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button: Button = findViewById(R.id.button)
val textView: TextView = findViewById(R.id.textView)
button.setOnClickListener {
textView.text = "Hello, Espresso!"
}
}
}
3. Write the Espresso Test: Create a new test class named MainActivityTest
in the androidTest
directory:
// src/androidTest/java/com/example/espresso/MainActivityTest.kt
package com.example.espresso
import androidx.test.ext.junit.rules.ActivityScenarioRule
import androidx.test.ext.junit.runners.AndroidJUnit4
import androidx.test.espresso.Espresso.onView
import androidx.test.espresso.action.ViewActions.click
import androidx.test.espresso.assertion.ViewAssertions.matches
import androidx.test.espresso.matcher.ViewMatchers.withId
import androidx.test.espresso.matcher.ViewMatchers.withText
import org.junit.Rule
import org.junit.Test
import org.junit.runner.RunWith
@RunWith(AndroidJUnit4::class)
class MainActivityTest {
@get:Rule
val activityRule = ActivityScenarioRule(MainActivity::class.java)
@Test
fun testButtonClick() {
// Perform a click on the button
onView(withId(R.id.button)).perform(click())
// Check if the text view displays the correct text
onView(withId(R.id.textView)).check(matches(withText("Hello, Espresso!")))
}
}
Understanding the Test Code
Let’s break down the test code to understand how Espresso works:
- ActivityScenarioRule: This rule launches the specified activity (in this case,
MainActivity
) before each test method annotated with@Test
, and closes it after the test completes. - onView(): This function finds a view in the current view hierarchy. You can specify the view using various matchers like
withId()
,withText()
, etc. - perform(): This function performs an action on the selected view. In our test, we use the
click()
action to simulate a button click. - check(): This function checks the state of the view using assertions. In our test, we use the
matches()
assertion to verify that the text view displays the expected text.
Advanced Espresso Features
Espresso offers several advanced features that make it a versatile and powerful testing framework. Let’s explore some of these features:
1. ViewMatchers
ViewMatchers are used to locate views in the current view hierarchy. Espresso provides a wide range of built-in matchers, including:
withId()
: Matches a view with the specified resource ID.withText()
: Matches a view with the specified text.withContentDescription()
: Matches a view with the specified content description.isDisplayed()
: Matches a view that is currently visible on the screen.
You can also create custom matchers to handle specific scenarios.
2. ViewActions
ViewActions are used to perform actions on the selected views. Some common actions include:
click()
: Simulates a click event.typeText()
: Types text into an input field.clearText()
: Clears the text in an input field.swipeLeft()
,swipeRight()
: Simulates swipe gestures.
3. ViewAssertions
ViewAssertions are used to verify the state of views. Some common assertions include:
matches()
: Checks if the view matches the specified matcher.doesNotExist()
: Checks if the view does not exist in the current view hierarchy.isDisplayed()
: Checks if the view is displayed on the screen.
4. Idling Resources
Espresso automatically handles synchronization with the UI thread, but there are cases where you might need to wait for background tasks (e.g., network requests) to complete. Idling Resources allow you to define custom conditions that Espresso should wait for before proceeding with the test.
Here’s an example of how to use Idling Resources:
// Define a custom IdlingResource
class MyIdlingResource : IdlingResource {
private var callback: IdlingResource.ResourceCallback? = null
override fun getName(): String = "MyIdlingResource"
override fun isIdleNow(): Boolean {
// Check if the background task is complete
val isIdle = ... // Your logic here
if (isIdle) {
callback?.onTransitionToIdle()
}
return isIdle
}
override fun registerIdleTransitionCallback(callback: IdlingResource.ResourceCallback?) {
this.callback = callback
}
}
// Use the IdlingResource in your test
val myIdlingResource = MyIdlingResource()
IdlingRegistry.getInstance().register(myIdlingResource)
onView(withId(R.id.button)).perform(click())
IdlingRegistry.getInstance().unregister(myIdlingResource)
Tips for Writing Effective Espresso Tests
- Keep Tests Independent: Ensure that each test is independent and does not rely on the state or outcome of other tests. This makes your tests more reliable and easier to debug.
- Use Descriptive Names: Give your test methods descriptive names that indicate what they are testing. This makes it easier to understand the purpose of each test at a glance.
- Mock External Dependencies: Use mocking frameworks like Mockito to mock external dependencies (e.g., network requests) in your tests. This allows you to isolate and test specific components without relying on external services.
- Run Tests on Real Devices: While emulators are useful for initial testing, it’s important to run your tests on real devices as well. Real devices provide a more accurate representation of how your app will perform in the hands of users.
Conclusion
Espresso is a powerful and flexible UI testing framework that can significantly improve the quality and reliability of your Android applications. By providing a simple API, automatic synchronization with the UI thread, and extensive support for matches, actions, and assertions, Espresso makes it easy to write effective and robust UI tests. Integrating Espresso into your development workflow ensures that your app’s user interface behaves as expected, delivering a seamless and bug-free experience to users.
As we have seen, setting up Espresso in your Android project is straightforward, and writing tests is both intuitive and efficient. By following best practices such as keeping tests independent, using descriptive names, mocking external dependencies, and running tests on real devices, you can make the most of Espresso’s capabilities.
Incorporating UI testing with Espresso into your development cycle not only helps catch bugs early but also enhances the overall maintainability of your codebase. With the combination of Kotlin’s expressive syntax and Espresso’s robust features, you can achieve a high level of confidence in your app’s UI, ultimately leading to higher quality applications and satisfied users.
Whether you are a seasoned developer or just starting with Android development, mastering Espresso will be a valuable addition to your skill set. Happy testing!