Audio and Video in Android with Kotlin, With the advent of mobile technology, multimedia applications have become a staple in the Android ecosystem. Whether it’s streaming music, playing videos, or displaying images, multimedia apps enhance user engagement and provide a richer experience. This blog will delve into the nitty-gritty of implementing audio and video playback in Android using Kotlin. By the end, you’ll have a comprehensive understanding of how to build robust media applications.
Table of Contents
Introduction – Audio and Video in Android
Media playback in Android is facilitated by various classes and frameworks provided by the Android SDK. Two primary components are MediaPlayer
for handling audio and video playback and ExoPlayer
, a more advanced, flexible, and customizable player.
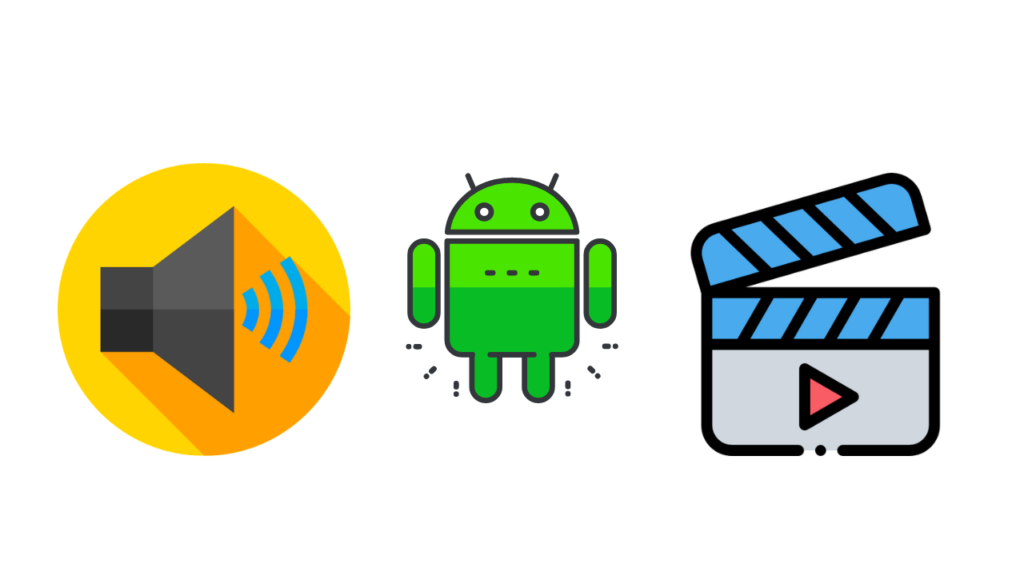
Setting Up Your Project
irst, ensure your Android project is set up for Kotlin. If you’re starting a new project, make sure to select Kotlin as the language. For existing projects, you can add Kotlin support by including the necessary Kotlin plugins and dependencies.
plugins {
id 'com.android.application'
id 'kotlin-android'
id 'kotlin-android-extensions'
}
android {
compileSdkVersion 30
defaultConfig {
applicationId "com.example.mediaplayback"
minSdkVersion 21
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
implementation 'androidx.core:core-ktx:1.3.2'
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'com.google.android.material:material:1.2.1'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
testImplementation 'junit:junit:4.13.1'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
Playing Audio with MediaPlayer
MediaPlayer
is a versatile class used to control playback of audio/video files and streams. Below is a basic example of playing an audio file from a URL.
Layout XML (activity_main.xml)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/playButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play" />
<Button
android:id="@+id/pauseButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Pause" />
<Button
android:id="@+id/stopButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stop" />
</LinearLayout>
package com.example.mediaplayback
import android.media.MediaPlayer
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
class MainActivity : AppCompatActivity() {
private lateinit var mediaPlayer: MediaPlayer
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val playButton: Button = findViewById(R.id.playButton)
val pauseButton: Button = findViewById(R.id.pauseButton)
val stopButton: Button = findViewById(R.id.stopButton)
mediaPlayer = MediaPlayer()
playButton.setOnClickListener {
mediaPlayer.reset()
mediaPlayer.setDataSource("https://www.example.com/audiofile.mp3")
mediaPlayer.prepare()
mediaPlayer.start()
}
pauseButton.setOnClickListener {
if (mediaPlayer.isPlaying) {
mediaPlayer.pause()
}
}
stopButton.setOnClickListener {
if (mediaPlayer.isPlaying) {
mediaPlayer.stop()
}
}
}
override fun onDestroy() {
super.onDestroy()
mediaPlayer.release()
}
}
Explanation
- Initializing MediaPlayer:
mediaPlayer = MediaPlayer()
- Setting Data Source:
mediaPlayer.setDataSource("https://www.example.com/audiofile.mp3")
- Preparing MediaPlayer:
mediaPlayer.prepare()
- Starting Playback:
mediaPlayer.start()
- Pausing Playback:
mediaPlayer.pause()
- Stopping Playback:
mediaPlayer.stop()
- Releasing Resources:
mediaPlayer.release()
Advanced Media Playback with ExoPlayer
ExoPlayer is an open-source project built on top of Android’s low-level media APIs. It is more robust and flexible than MediaPlayer
, making it ideal for complex media applications.
Adding ExoPlayer Dependency
Add the ExoPlayer dependency to your build.gradle
file:
gradleCopy codeimplementation 'com.google.android.exoplayer:exoplayer:2.14.0'
Layout XML (activity_main.xml)
xmlCopy code<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<com.google.android.exoplayer2.ui.PlayerView
android:id="@+id/playerView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/playButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play" />
<Button
android:id="@+id/pauseButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Pause" />
<Button
android:id="@+id/stopButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stop" />
</LinearLayout>
Kotlin Activity (MainActivity.kt)
kotlinCopy codepackage com.example.mediaplayback
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.google.android.exoplayer2.ExoPlayer
import com.google.android.exoplayer2.MediaItem
import com.google.android.exoplayer2.ui.PlayerView
import android.widget.Button
class MainActivity : AppCompatActivity() {
private lateinit var player: ExoPlayer
private lateinit var playerView: PlayerView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
playerView = findViewById(R.id.playerView)
val playButton: Button = findViewById(R.id.playButton)
val pauseButton: Button = findViewById(R.id.pauseButton)
val stopButton: Button = findViewById(R.id.stopButton)
player = ExoPlayer.Builder(this).build()
playerView.player = player
val mediaItem = MediaItem.fromUri("https://www.example.com/videofile.mp4")
player.setMediaItem(mediaItem)
playButton.setOnClickListener {
player.prepare()
player.play()
}
pauseButton.setOnClickListener {
player.pause()
}
stopButton.setOnClickListener {
player.stop()
}
}
override fun onDestroy() {
super.onDestroy()
player.release()
}
}
Explanation
- Initializing ExoPlayer:
player = ExoPlayer.Builder(this).build()
- Setting PlayerView:
playerView.player = player
- Creating MediaItem:
MediaItem.fromUri("https://www.example.com/videofile.mp4")
- Setting MediaItem:
player.setMediaItem(mediaItem)
- Preparing Player:
player.prepare()
- Starting Playback:
player.play()
- Pausing Playback:
player.pause()
- Stopping Playback:
player.stop()
- Releasing Resources:
player.release()
Handling Lifecycle Events
Properly handling lifecycle events is crucial for a smooth user experience. For both MediaPlayer
and ExoPlayer
, releasing resources in the onDestroy
method ensures no memory leaks.
Example with ExoPlayer
kotlinCopy codeoverride fun onPause() {
super.onPause()
player.pause()
}
override fun onResume() {
super.onResume()
player.play()
}
override fun onDestroy() {
super.onDestroy()
player.release()
}
Handling Errors and State Changes
ExoPlayer is an open-source project built on top of Android’s low-level media APIs. It is more robust and flexible than MediaPlayer
, making it ideal for complex media applications.
Adding ExoPlayer Dependency
Add the ExoPlayer dependency to your build.gradle
file:
implementation 'com.google.android.exoplayer:exoplayer:2.14.0'
Layout XML (activity_main.xml)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<com.google.android.exoplayer2.ui.PlayerView
android:id="@+id/playerView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/playButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play" />
<Button
android:id="@+id/pauseButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Pause" />
<Button
android:id="@+id/stopButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stop" />
</LinearLayout>
package com.example.mediaplayback
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.google.android.exoplayer2.ExoPlayer
import com.google.android.exoplayer2.MediaItem
import com.google.android.exoplayer2.ui.PlayerView
import android.widget.Button
class MainActivity : AppCompatActivity() {
private lateinit var player: ExoPlayer
private lateinit var playerView: PlayerView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
playerView = findViewById(R.id.playerView)
val playButton: Button = findViewById(R.id.playButton)
val pauseButton: Button = findViewById(R.id.pauseButton)
val stopButton: Button = findViewById(R.id.stopButton)
player = ExoPlayer.Builder(this).build()
playerView.player = player
val mediaItem = MediaItem.fromUri("https://www.example.com/videofile.mp4")
player.setMediaItem(mediaItem)
playButton.setOnClickListener {
player.prepare()
player.play()
}
pauseButton.setOnClickListener {
player.pause()
}
stopButton.setOnClickListener {
player.stop()
}
}
override fun onDestroy() {
super.onDestroy()
player.release()
}
}
Explanation
- Initializing ExoPlayer:
player = ExoPlayer.Builder(this).build()
- Setting PlayerView:
playerView.player = player
- Creating MediaItem:
MediaItem.fromUri("https://www.example.com/videofile.mp4")
- Setting MediaItem:
player.setMediaItem(mediaItem)
- Preparing Player:
player.prepare()
- Starting Playback:
player.play()
- Pausing Playback:
player.pause()
- Stopping Playback:
player.stop()
- Releasing Resources:
player.release()
Handling Lifecycle Events
Properly handling lifecycle events is crucial for a smooth user experience. For both MediaPlayer
and ExoPlayer
, releasing resources in the onDestroy
method ensures no memory leaks.
Example with ExoPlayer
override fun onPause() {
super.onPause()
player.pause()
}
override fun onResume() {
super.onResume()
player.play()
}
override fun onDestroy() {
super.onDestroy()
player.release()
}
Handling Errors and State Changes
Handling errors and state changes is essential for a resilient media playback application. Both MediaPlayer
and ExoPlayer
provide listeners to monitor playback state and errors.
ExoPlayer Error Handling
player.addListener(object : Player.Listener {
override fun onPlayerError(error: ExoPlaybackException) {
// Handle error
}
override fun onPlaybackStateChanged(state: Int) {
when (state) {
Player.STATE_IDLE -> {
// The player is idle and not ready to play
}
Player.STATE_BUFFERING -> {
// The player is buffering
}
Player.STATE_READY -> {
// The player is ready to play
}
Player.STATE_ENDED -> {
// The player has finished playing
}
}
}
})
MediaPlayer Error Handling
mediaPlayer.setOnErrorListener { mp, what, extra ->
// Handle error
true
}
mediaPlayer.setOnPreparedListener {
// Ready to play
}
Conclusion
Building media playback applications in Android using Kotlin is both straightforward and powerful with the right tools. MediaPlayer
is perfect for simple use cases, while ExoPlayer
offers advanced features for more complex scenarios. By understanding the basics and advanced capabilities of these players, you can create rich, multimedia experiences for your users.
To further enhance your applications, consider exploring additional features such as streaming from different sources, handling DRM-protected content, and integrating with other Android components like notifications and background services.
With this foundation, you’re well-equipped to start building your own media playback applications in Android using Kotlin. Happy coding!