In the realm of modern mobile applications, sensors play a pivotal role in enhancing user experiences. Among these sensors, the accelerometer and gyroscope are crucial for detecting motion and orientation. Whether you’re developing a fitness app that tracks physical activity or a game that requires precise motion control, understanding how to use these sensors in Android with Kotlin is essential. In this comprehensive guide, we’ll explore the accelerometer and gyroscope, how they work, and how to implement them in an Android application using Kotlin.
Table of Contents
Introduction to Accelerometer and Gyroscope in Android
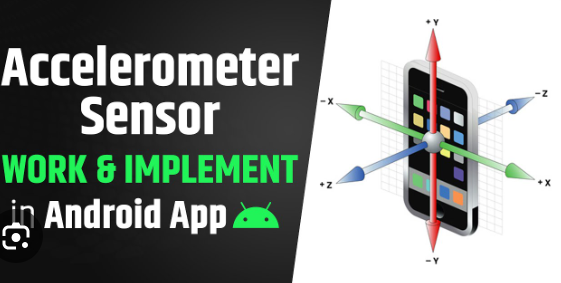
What is an Accelerometer?
An accelerometer is a sensor that measures the acceleration force acting on a device. It can detect changes in movement and orientation by measuring the rate of change of velocity. Accelerometers are commonly used to detect device orientation, shake gestures, and linear motion.
What is a Gyroscope?
A gyroscope, on the other hand, measures the rate of rotation around the device’s three primary axes (x, y, and z). It provides precise information about the device’s orientation and rotational movement, which is crucial for applications that require high accuracy in motion detection, such as augmented reality (AR) and gaming applications.
Setting Up Sensor Management in Android
To work with sensors in an Android application, you’ll need to use the SensorManager
class, which provides access to the device’s sensors. You’ll also need to implement the SensorEventListener
interface to receive sensor event updates.
Adding Dependencies
First, ensure that you have the necessary dependencies in your build.gradle
file:
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
implementation 'androidx.appcompat:appcompat:1.3.0'
implementation 'androidx.core:core-ktx:1.6.0'
}
Requesting Permissions
Depending on your use case, you might need to request certain permissions. For most sensor-based applications, no special permissions are required. However, if you are using sensors in conjunction with other features like location services, ensure you request the necessary permissions in your AndroidManifest.xml
file.
Implementing Accelerometer in Kotlin
Let’s start by implementing the accelerometer. We’ll create an application that detects and displays the device’s orientation based on accelerometer data.
Step 1: Set Up the UI
Create a simple layout with TextViews
to display the accelerometer data. Add the following code to your res/layout/activity_main.xml
file:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/textViewX"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="X: " />
<TextView
android:id="@+id/textViewY"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Y: " />
<TextView
android:id="@+id/textViewZ"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Z: " />
</LinearLayout>
Step 2: Implement the Accelerometer Logic
In your MainActivity.kt
, set up the SensorManager
and implement the SensorEventListener
to receive accelerometer updates:
import android.hardware.Sensor
import android.hardware.SensorEvent
import android.hardware.SensorEventListener
import android.hardware.SensorManager
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import android.widget.TextView
class MainActivity : AppCompatActivity(), SensorEventListener {
private lateinit var sensorManager: SensorManager
private var accelerometer: Sensor? = null
private lateinit var textViewX: TextView
private lateinit var textViewY: TextView
private lateinit var textViewZ: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
textViewX = findViewById(R.id.textViewX)
textViewY = findViewById(R.id.textViewY)
textViewZ = findViewById(R.id.textViewZ)
sensorManager = getSystemService(SENSOR_SERVICE) as SensorManager
accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER)
}
override fun onResume() {
super.onResume()
accelerometer?.also { acc ->
sensorManager.registerListener(this, acc, SensorManager.SENSOR_DELAY_NORMAL)
}
}
override fun onPause() {
super.onPause()
sensorManager.unregisterListener(this)
}
override fun onSensorChanged(event: SensorEvent?) {
if (event?.sensor?.type == Sensor.TYPE_ACCELEROMETER) {
val x = event.values[0]
val y = event.values[1]
val z = event.values[2]
textViewX.text = "X: $x"
textViewY.text = "Y: $y"
textViewZ.text = "Z: $z"
}
}
override fun onAccuracyChanged(sensor: Sensor?, accuracy: Int) {
// Do something if sensor accuracy changes
}
}
Explanation
SensorManager
and get the default accelerometer sensor. Lifecycle Methods: In onResume
, we register the sensor listener to start receiving updates. In onPause
, we unregister the listener to stop receiving updates when the activity is not in the foreground.SensorEventListener: We implement the onSensorChanged
method to receive sensor data and update the UI.Implementing Gyroscope in Kotlin
Next, let’s implement the gyroscope. We’ll create an application that detects and displays the device’s rotational movement.
Step 1: Set Up the UI
Add TextViews
to display the gyroscope data in your res/layout/activity_main.xml
file:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/textViewGyroX"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Gyro X: " />
<TextView
android:id="@+id/textViewGyroY"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Gyro Y: " />
<TextView
android:id="@+id/textViewGyroZ"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Gyro Z: " />
</LinearLayout>
Step 2: Implement the Gyroscope Logic
In your MainActivity.kt
, set up the SensorManager
and implement the SensorEventListener
to receive gyroscope updates:
import android.hardware.Sensor
import android.hardware.SensorEvent
import android.hardware.SensorEventListener
import android.hardware.SensorManager
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import android.widget.TextView
class MainActivity : AppCompatActivity(), SensorEventListener {
private lateinit var sensorManager: SensorManager
private var gyroscope: Sensor? = null
private lateinit var textViewGyroX: TextView
private lateinit var textViewGyroY: TextView
private lateinit var textViewGyroZ: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
textViewGyroX = findViewById(R.id.textViewGyroX)
textViewGyroY = findViewById(R.id.textViewGyroY)
textViewGyroZ = findViewById(R.id.textViewGyroZ)
sensorManager = getSystemService(SENSOR_SERVICE) as SensorManager
gyroscope = sensorManager.getDefaultSensor(Sensor.TYPE_GYROSCOPE)
}
override fun onResume() {
super.onResume()
gyroscope?.also { gyro ->
sensorManager.registerListener(this, gyro, SensorManager.SENSOR_DELAY_NORMAL)
}
}
override fun onPause() {
super.onPause()
sensorManager.unregisterListener(this)
}
override fun onSensorChanged(event: SensorEvent?) {
if (event?.sensor?.type == Sensor.TYPE_GYROSCOPE) {
val x = event.values[0]
val y = event.values[1]
val z = event.values[2]
textViewGyroX.text = "Gyro X: $x"
textViewGyroY.text = "Gyro Y: $y"
textViewGyroZ.text = "Gyro Z: $z"
}
}
override fun onAccuracyChanged(sensor: Sensor?, accuracy: Int) {
// Do something if sensor accuracy changes
}
}
Explanation
Initialization: We initialize the SensorManager
and get the default gyroscope sensor.
Lifecycle Methods: In onResume
, we register the sensor listener to start receiving updates. In onPause
, we unregister the listener to stop receiving updates when the activity is not in the foreground.
SensorEventListener: We implement the
Conclusion
Accelerometers and gyroscopes are powerful sensors that provide valuable data for developing responsive and interactive Android applications. By understanding and utilizing these sensors, you can create apps that can detect motion, orientation, and rotation, opening up a world of possibilities for innovative features and user experiences.
In this guide, we’ve explored the basics of accelerometers and gyroscopes, how they work, and how to implement them in an Android application using Kotlin. We walked through setting up the SensorManager
, registering and unregistering sensor listeners, and handling sensor events to update the UI with real-time sensor data.
Key Takeaways
- Accelerometers measure the acceleration force acting on the device, which is useful for detecting linear motion and orientation changes.
- Gyroscopes measure the rate of rotation around the device’s axes, providing precise information about rotational movement and orientation.
- SensorManager and SensorEventListener are crucial components for working with sensors in Android, allowing you to manage sensor data efficiently.
- Lifecycle Management: It’s important to register sensor listeners in
onResume
and unregister them inonPause
to ensure efficient use of system resources and prevent battery drain. - UI Updates: Handle sensor events to update the UI in real-time, providing users with immediate feedback based on their interactions with the device.
Practical Applications
The knowledge of using accelerometers and gyroscopes can be applied to various types of applications, such as:
- Fitness and Health Apps: Track physical activity and exercise routines.
- Gaming: Create immersive and interactive game controls.
- Augmented Reality (AR): Enhance AR experiences by detecting precise device movements.
- User Interface Enhancements: Implement features like shake-to-refresh or device orientation-based changes.
By leveraging these sensors, you can create applications that respond intuitively to user movements and provide a richer, more engaging user experience. As you continue to explore and experiment with these sensors, you’ll uncover even more creative ways to integrate motion and orientation detection into your Android applications.
Remember to always test your applications on real devices to ensure accurate sensor data and optimal performance. With the right approach and attention to detail, you can harness the full potential of accelerometers and gyroscopes to build innovative and responsive Android apps.