In today’s mobile-first world, location-based services have become integral to various applications. Whether you’re developing a navigation app, a food delivery service, or a travel planner, integrating GPS and Maps can significantly enhance user experience. This blog will guide you through creating an Android application that utilizes GPS and Google Maps using Kotlin.
Table of Contents
Introduction GPS and Maps in an Android
GPS (Global Positioning System) and maps are fundamental components of location-based services. By leveraging these technologies, developers can create applications that offer real-time navigation, location tracking, and much more.
In this tutorial, we’ll build a simple Android application that displays the user’s current location on a Google Map. We will cover how to set up your project, add Google Maps, get the user’s location, and display it on the map.
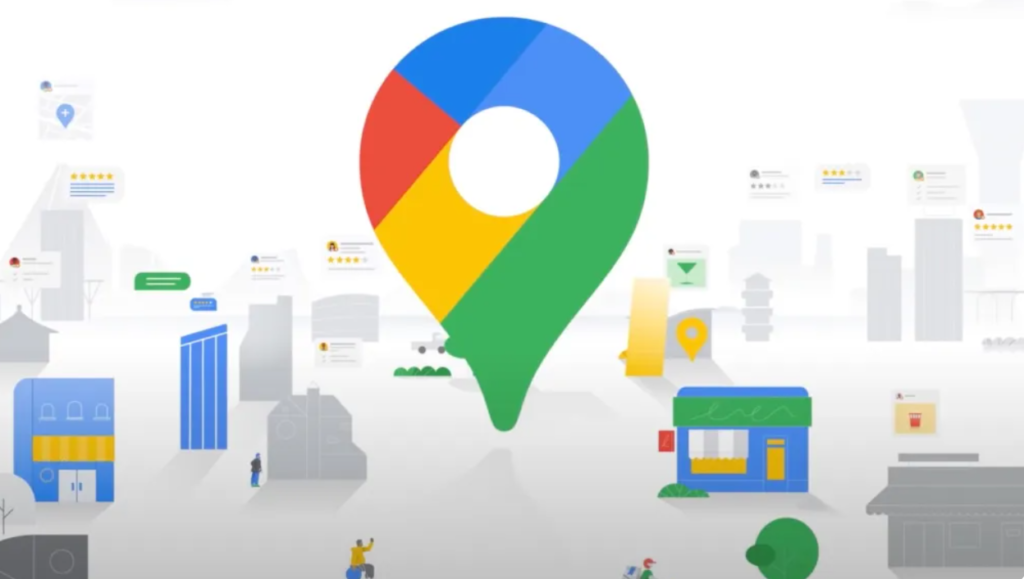
Setting Up Your Project
First, let’s set up a new Android project in Android Studio.
- Create a New Project
- Open Android Studio and select “New Project.”
- Choose the “Empty Activity” template.
- Name your project, choose Kotlin as the language, and click “Finish.”
- Add Google Play Services To use Google Maps, you need to add the Google Play Services dependency to your project. Open your
build.gradle (app)
file and add the following line inside thedependencies
block:
implementation 'com.google.android.gms:play-services-maps:18.0.2'
3. Obtain an API Key To use Google Maps, you need an API key. Follow these steps to get one:
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Navigate to the “Credentials” page and create an API key.
- Enable the “Maps SDK for Android” API for your project.
4. Add the API Key to Your Project Open your AndroidManifest.xml
file and add the following lines inside the <application>
tag:
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_API_KEY_HERE" />
Replace YOUR_API_KEY_HERE
with your actual API key.
Adding Google Maps to Your Application
Now that your project is set up, let’s add a Google Map to your application.
- Create a Map Activity In your
res/layout
directory, create a new layout file namedactivity_maps.xml
and add the following XML code:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MapsActivity">
<com.google.android.gms.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
2. Create a New Activity Create a new activity named MapsActivity
and update it as follows:
package com.example.yourapp
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.google.android.gms.maps.CameraUpdateFactory
import com.google.android.gms.maps.GoogleMap
import com.google.android.gms.maps.OnMapReadyCallback
import com.google.android.gms.maps.SupportMapFragment
import com.google.android.gms.maps.model.LatLng
import com.google.android.gms.maps.model.MarkerOptions
class MapsActivity : AppCompatActivity(), OnMapReadyCallback {
private lateinit var map: GoogleMap
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_maps)
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
val mapFragment = supportFragmentManager
.findFragmentById(R.id.mapView) as SupportMapFragment
mapFragment.getMapAsync(this)
}
override fun onMapReady(googleMap: GoogleMap) {
map = googleMap
// Add a marker in Sydney and move the camera
val sydney = LatLng(-34.0, 151.0)
map.addMarker(MarkerOptions().position(sydney).title("Marker in Sydney"))
map.moveCamera(CameraUpdateFactory.newLatLng(sydney))
}
}
Getting User Location
To get the user’s current location, we need to use the FusedLocationProviderClient
provided by Google Play Services.
- Add Location Dependency Open your
build.gradle (app)
file and add the following line inside thedependencies
block:
implementation 'com.google.android.gms:play-services-location:21.0.1'
2. Request Location Permissions Open your AndroidManifest.xml
file and add the following permissions:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
3. Initialize FusedLocationProviderClient Update your MapsActivity
to initialize the FusedLocationProviderClient
and request location permissions:
package com.example.yourapp
import android.Manifest
import android.content.pm.PackageManager
import android.os.Bundle
import androidx.core.app.ActivityCompat
import androidx.core.content.ContextCompat
import com.google.android.gms.location.FusedLocationProviderClient
import com.google.android.gms.location.LocationServices
import com.google.android.gms.maps.CameraUpdateFactory
import com.google.android.gms.maps.GoogleMap
import com.google.android.gms.maps.OnMapReadyCallback
import com.google.android.gms.maps.SupportMapFragment
import com.google.android.gms.maps.model.LatLng
import com.google.android.gms.maps.model.MarkerOptions
class MapsActivity : AppCompatActivity(), OnMapReadyCallback {
private lateinit var map: GoogleMap
private lateinit var fusedLocationClient: FusedLocationProviderClient
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_maps)
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
val mapFragment = supportFragmentManager
.findFragmentById(R.id.mapView) as SupportMapFragment
mapFragment.getMapAsync(this)
fusedLocationClient = LocationServices.getFusedLocationProviderClient(this)
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(
this,
arrayOf(Manifest.permission.ACCESS_FINE_LOCATION),
1
)
}
}
override fun onMapReady(googleMap: GoogleMap) {
map = googleMap
// Check if location permission is granted
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
== PackageManager.PERMISSION_GRANTED) {
map.isMyLocationEnabled = true
fusedLocationClient.lastLocation.addOnSuccessListener { location ->
if (location != null) {
val currentLatLng = LatLng(location.latitude, location.longitude)
map.addMarker(MarkerOptions().position(currentLatLng).title("You are here"))
map.moveCamera(CameraUpdateFactory.newLatLngZoom(currentLatLng, 15f))
}
}
}
}
override fun onRequestPermissionsResult(
requestCode: Int,
permissions: Array<out String>,
grantResults: IntArray
) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
if (requestCode == 1 && grantResults.isNotEmpty() && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted
onMapReady(map)
}
}
}
Displaying User Location on the Map
The FusedLocationProviderClient
provides the last known location of the device, which we can use to display the user’s location on the map.
- Check Location Permission Ensure that the location permission is granted before enabling the location layer on the map.
- Get Last Known Location Use the
getLastLocation
method ofFusedLocationProviderClient
to get the last known location of the device. - Add a Marker for the Current Location Once the location is obtained, add a marker on the map to indicate the user’s current location.
Handling Permissions
Handling permissions is a crucial aspect of working with location services. Users need to grant permission for your app to access their location.
- Request Permissions Request location permissions in the
onCreate
method of your activity. - Handle Permission Result Override the
onRequestPermissionsResult
method to handle the result of the permission request.
Conclusion
In this blog, we’ve covered the basics of integrating GPS and Google Maps into an Android application using Kotlin. We’ve set up a new project, added Google Maps, obtained the user’s location, and displayed it on the map. With these fundamentals, you can build more complex location-based features into your applications.
Integrating GPS and Maps can open up a world of possibilities for your app, from simple location tracking to complex navigation systems. Experiment with different features and build something amazing!
Remember to handle permissions carefully and respect user privacy by providing clear information on why your app needs access to their location. Happy coding!